Today I learned about a handy utility called eslint-config-prettier-check
which I saw referenced in this GitHub issue.
I was editing a .vue
component in Visual Studio Code. I had a code section with red underlines, generally indicating that eslint was not happy with something. I could see it was a formatting issue, so I used eslint --fix
to solve the issue and re-format the code. But then that triggered a new lint problem where Prettier was now unhappy with how eslint had formatted the code. The difference between the two is small, and involves a couple of indented spaces. See the images below for both examples.
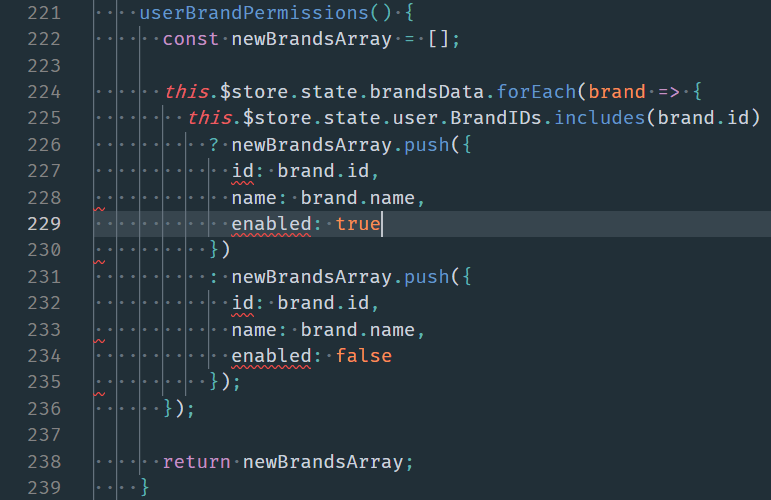
eslint Formatted
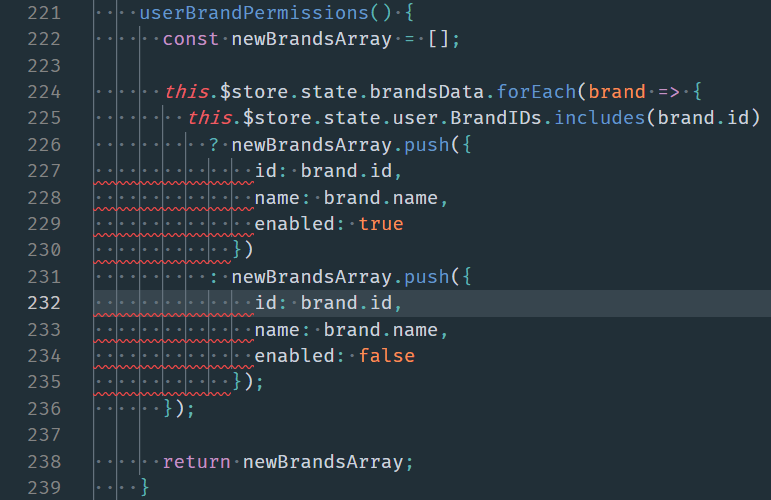